目次
はじめに
「ポップアップで情報を表示したいけど、実装方法がわからない…」
「アニメーションをつけたモーダルの作り方がわからない…」
そんな悩みを持つ方も多いのではないでしょうか?
この記事では、モーダルウィンドウの基本から実践的な実装方法まで、初心者にもわかりやすく解説します。
コピペするだけで実装できるコードも用意していますので、ぜひ最後までご覧ください。
詳しく解説していますので、コードだけほしい方は最後の「完成コード」までスクロールしてください。
見本
今回は下記のようなモーダルウィンドウを作っていきます。
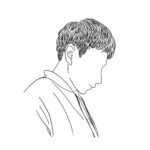
「モーダルを開くボタン」を押してみてください!
See the Pen Untitled by ryoma (@hwjgdjpk-the-decoder) on CodePen.
ポイントは下記になります。
- 開くボタンをクリックするとモーダルウィンドウが表示される
- 背景がオーバーレイで暗くなる
- ×ボタンまたは背景をクリックすると閉じる
- スムーズなアニメーション効果付き
モーダルウィンドウの見た目の制作
HTML
<!-- モーダルウィンドウを開くボタン -->
<button class="modal-open js-modal-open">モーダルを開く</button>
<!-- モーダルウィンドウ -->
<div class="modal js-modal">
<div class="modal-overlay js-modal-close"></div>
<div class="modal-content">
<div class="modal-content__inner">
<h2 class="modal-content__title">モーダルタイトル</h2>
<p class="modal-content__text">
ここにモーダルの内容が入ります。
</p>
<button class="modal-close js-modal-close">×</button>
</div>
</div>
</div>
まずはHTMLですが、大きく分けて以下の2つの構造になっています。
- モーダルを開くボタン
- modal-open: スタイリングのためのクラス
- js-modal-open: JavaScriptで操作するためのクラス
- モーダルウィンドウの本体
- modal: スタイリングのためのクラス
- js-modal: JavaScriptで操作するためのクラス
- modal-overlay: 背景を暗くするオーバーレイ部分
- modal-content: モーダルの中身を表示する白い部分
CSS
/*=========================================
#modal
=========================================*/
.modal {
visibility: hidden;
opacity: 0;
position: fixed;
top: 0;
left: 0;
z-index: 100;
width: 100%;
height: 100vh;
transition: opacity .3s, visibility .3s;
}
.modal.is-active {
visibility: visible;
opacity: 1;
}
/* オーバーレイ */
.modal-overlay {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, .6);
cursor: pointer;
}
/* モーダルコンテンツ */
.modal-content {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
max-width: 600px;
width: 90%;
background-color: #fff;
border-radius: 8px;
}
.modal-content__inner {
padding: 30px;
position: relative;
}
/* 閉じるボタン */
.modal-close {
position: absolute;
top: 10px;
right: 10px;
width: 30px;
height: 30px;
border: none;
background: transparent;
cursor: pointer;
font-size: 24px;
}
主要なポイントを以下に解説します。
- モーダル全体のスタイリング
- visibility と opacity で表示/非表示を制御
- position: fixed で画面全体に固定
- transition でアニメーション効果を追加
- オーバーレイ部分
- background-color: rgba() で半透明の黒背景
- cursor: pointer でクリックできることを示す
- コンテンツ部分
- position: absolute と transform で中央配置
- max-width と width で適切なサイズ設定
モーダルウィンドウの動きの制作
見た目が完成したので、次は動きをつけてモーダルの開閉ができるようにしていきます。
ポイントをおさらいすると以下のようになります。
- 開くボタンをクリックするとモーダルが表示される
- ×ボタンをクリックすると閉じる
- オーバーレイ(背景)をクリックしても閉じる
- スムーズなアニメーション効果がつく
JavaScriptの完成コード
/*=========================================
#モーダルウィンドウ
=========================================*/
const modal = document.querySelector(".js-modal");
const modalOpen = document.querySelector(".js-modal-open");
const modalClose = document.querySelectorAll(".js-modal-close");
// モーダルを開く
modalOpen.addEventListener('click', () => {
modal.classList.add('is-active');
});
// モーダルを閉じる
modalClose.forEach(closeButton => {
closeButton.addEventListener('click', () => {
modal.classList.remove('is-active');
});
});
上記コードは、モーダルウィンドウの開閉を制御するためのものです。
主要な部分を解説します。
- 要素の取得
const modal = document.querySelector(".js-modal");
const modalOpen = document.querySelector(".js-modal-open");
const modalClose = document.querySelectorAll(".js-modal-close");
モーダル本体、開くボタン、閉じるボタン(複数)を取得します
- 開く処理
modalOpen.addEventListener('click', () => {
modal.classList.add('is-active');
});
開くボタンがクリックされたときにis-active
クラスを追加
- 閉じる処理
modalClose.forEach(closeButton => {
closeButton.addEventListener('click', () => {
modal.classList.remove('is-active');
});
});
- 閉じるボタンと背景の両方に対してクリックイベントを設定
- クリックされたときに
is-active
クラスを削除
完成コード
以下は完成後のコードです:
HTML
<!-- モーダルを開くボタン -->
<button class="modal-open js-modal-open">モーダルを開く</button>
<!-- モーダルウィンドウ -->
<div class="modal js-modal">
<div class="modal-overlay js-modal-close"></div>
<div class="modal-content">
<div class="modal-content__inner">
<h2 class="modal-content__title">モーダルタイトル</h2>
<p class="modal-content__text">
ここにモーダルの内容が入ります。
</p>
<button class="modal-close js-modal-close">×</button>
</div>
</div>
</div>
CSS
/*=========================================
#modal
=========================================*/
.modal {
visibility: hidden;
opacity: 0;
position: fixed;
top: 0;
left: 0;
z-index: 100;
width: 100%;
height: 100vh;
transition: opacity .3s, visibility .3s;
}
.modal.is-active {
visibility: visible;
opacity: 1;
}
/* オーバーレイ */
.modal-overlay {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, .6);
cursor: pointer;
}
/* モーダルコンテンツ */
.modal-content {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
max-width: 600px;
width: 90%;
background-color: #fff;
border-radius: 8px;
}
.modal-content__inner {
padding: 30px;
position: relative;
}
/* 閉じるボタン */
.modal-close {
position: absolute;
top: 10px;
right: 10px;
width: 30px;
height: 30px;
border: none;
background: transparent;
cursor: pointer;
font-size: 24px;
}
JavaScript
/*=========================================
#モーダルウィンドウ
=========================================*/
const modal = document.querySelector(".js-modal");
const modalOpen = document.querySelector(".js-modal-open");
const modalClose = document.querySelectorAll(".js-modal-close");
modalOpen.addEventListener('click', () => {
modal.classList.add('is-active');
});
modalClose.forEach(closeButton => {
closeButton.addEventListener('click', () => {
modal.classList.remove('is-active');
});
});
まとめ
今回は簡単にモーダルウィンドウを作る方法を詳しく解説しました。
モーダルウィンドウは使う機会も非常に多いので、この記事をブックマークして、すぐに見返すことができるようにしておくと便利です。
また、このブログでは他にもコーディングに関する記事を投稿していますので、あわせて参考にしてみてください。