はじめに
HTML/CSSは理解できたものの、JavaScriptでの実装に悩んでいませんか?特にローディングアニメーションは、多くのWebサイトで使用される重要な要素ですが、初心者にとっては実装のハードルが高く感じられるものです。
実は、基本的なローディングアニメーションは数行のコードで実装できます。この記事では、実務でもすぐに活用できる具体的な実装方法を、初心者の方にも分かりやすく解説していきます。
5分程度の短時間で、コピー&ペーストだけで動作確認できるサンプルコードも用意しました。通勤時間や休憩時間にさっと読んで、すぐに実践できる内容となっています。
見本
今回は以下のようなものを作成していきます。
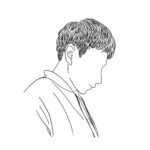
ロードしてみてください
See the Pen Untitled by ryoma (@hwjgdjpk-the-decoder) on CodePen.
ローディングアニメーションの実装
1. HTML
まずは、HTMLの構造を作成します。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>シンプルなローディングアニメーション</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="loading" id="loading">
<div class="spinner"></div>
<p class="loading-text">Loading...</p>
</div>
<div class="content" id="content">
<!-- メインコンテンツがここに入ります -->
<h1>コンテンツが表示されました!</h1>
</div>
<script src="script.js"></script>
</body>
</html>
このHTMLでは、主に4つの重要な要素があります。
- ローディングコンテナ – 画面全体を覆うオーバーレイとして機能し、JavaScriptで制御します
- スピナー要素 – CSSアニメーションの中心となる回転する要素です
- ローディングテキスト – ユーザーへのフィードバックとアクセシビリティのために配置します
- コンテンツコンテナ – 実際のページコンテンツを格納し、ローディング完了後に表示します
この構造により、ローディング中の表示とコンテンツの切り替えをスムーズに行うことができます。
2. CSS
.loading {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: #fff;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
z-index: 1000;
}
.spinner {
width: 50px;
height: 50px;
border: 5px solid #f3f3f3;
border-top: 5px solid #3498db;
border-radius: 50%;
animation: spin 1s linear infinite;
}
@keyframes spin {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
.loading-text {
margin-top: 15px;
font-family: Arial, sans-serif;
color: #333;
}
.content {
display: none;
}
.show {
display: block;
}
このCSSコードの主要なポイントは以下の通りです。
ローディング画面のスタイル(.loading)
position: fixed
とtop/left: 0
で画面全体を覆うwidth/height: 100%
で画面いっぱいに広がるdisplay: flex
と各種配置プロパティで中央揃えを実現z-index: 1000
で最前面に表示
スピナーのスタイル(.spinner)
- 円形の形状を
width/height
とborder-radius
で作成 - グレーの円周に青いアクセントを
border
で表現 animation: spin
で回転アニメーションを適用
アニメーションの定義(@keyframes)
- 0度から360度までの回転を1秒間で繰り返し
linear
で一定速度の滑らかな動きを実現
コンテンツの表示制御
- 初期状態では
display: none
で非表示 .show
クラスでdisplay: block
に切り替え
3. JavaScript
document.addEventListener('DOMContentLoaded', function() {
const loading = document.getElementById('loading');
const content = document.getElementById('content')
// ローディング画面を表示する関数
function showLoading() {
loading.style.display = 'flex';
content.classList.remove('show');
}
// ローディング画面を非表示にする関数
function hideLoading() {
loading.style.display = 'none';
content.classList.add('show');
}
// 2秒後にローディング画面を非表示にする
setTimeout(hideLoading, 2000);
});
このJavaScriptコードでは、ローディング表示を制御する基本的な機能を実装しています。
主要なポイントは以下の通りです。
初期設定
- DOMContentLoaded イベントで DOM 読み込み完了を待機
- 必要な要素を getElementById で取得
制御関数
- showLoading関数
- ローディング画面を表示
- コンテンツを非表示に
hideLoading関数
- ローディング画面を非表示
- コンテンツを表示
タイミング制御
- setTimeout で2秒後に自動的に切り替え
- 実際のアプリケーションでは、データ読み込みなどの完了を待つように変更可能
カスタマイズ
ローディングアニメーションのカスタマイズパターン集を紹介させていただきます。
どれもコピペで作成可能ですので、参考にしてみてください。
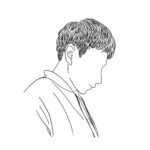
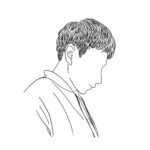
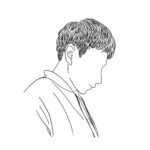
今後も逐次追加していきます!
1. パルス型ローディング
See the Pen Untitled by ryoma (@hwjgdjpk-the-decoder) on CodePen.
HTML
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>パルス型ローディング</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="loading" id="loading">
<div class="pulse-container">
<div class="pulse-bubble pulse-bubble-1"></div>
<div class="pulse-bubble pulse-bubble-2"></div>
<div class="pulse-bubble pulse-bubble-3"></div>
</div>
<p class="loading-text">Loading...</p>
</div>
<div class="content" id="content">
<h1>コンテンツが表示されました!</h1>
</div>
<script src="script.js"></script>
</body>
</html>
CSS
.loading {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(255, 255, 255, 0.95);
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
z-index: 1000;
}
.pulse-container {
display: flex;
justify-content: space-between;
width: 100px;
}
.pulse-bubble {
width: 20px;
height: 20px;
border-radius: 50%;
background-color: #3498db;
}
.pulse-bubble-1 {
animation: pulse 0.4s ease 0s infinite alternate;
}
.pulse-bubble-2 {
animation: pulse 0.4s ease 0.2s infinite alternate;
}
.pulse-bubble-3 {
animation: pulse 0.4s ease 0.4s infinite alternate;
}
@keyframes pulse {
from {
opacity: 1;
transform: scale(1);
}
to {
opacity: 0.25;
transform: scale(0.75);
}
}
.content {
display: none;
}
.show {
display: block;
}
JavaScript
document.addEventListener('DOMContentLoaded', function() {
const loading = document.getElementById('loading');
const content = document.getElementById('content');
// ローディング画面を表示する関数
function showLoading() {
loading.style.display = 'flex';
content.classList.remove('show');
}
// ローディング画面を非表示にする関数
function hideLoading() {
loading.style.display = 'none';
content.classList.add('show');
}
// 3秒後にローディング画面を非表示にする
setTimeout(hideLoading, 3000);
});
2. プログレスバー型ローディング
See the Pen Untitled by ryoma (@hwjgdjpk-the-decoder) on CodePen.
HTML
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>プログレスバー型ローディング</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="loading" id="loading">
<div class="progress-container">
<div class="progress-bar" id="progress"></div>
</div>
<p class="loading-text">Loading: <span id="progress-text">0%</span></p>
</div>
<div class="content" id="content">
<h1>コンテンツが表示されました!</h1>
</div>
<script src="script.js"></script>
</body>
</html>
CSS
.loading {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(255, 255, 255, 0.95);
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
z-index: 1000;
}
.progress-container {
width: 300px;
height: 20px;
background: #f0f0f0;
border-radius: 10px;
overflow: hidden;
}
.progress-bar {
width: 0%;
height: 100%;
background: linear-gradient(90deg, #3498db, #2ecc71);
transition: width 0.3s ease;
}
.content {
display: none;
}
.show {
display: block;
}
JavaScript
document.addEventListener('DOMContentLoaded', function() {
const loading = document.getElementById('loading');
const content = document.getElementById('content');
const progressBar = document.getElementById('progress');
const progressText = document.getElementById('progress-text');
let progress = 0;
function updateProgress() {
if (progress < 100) {
progress += Math.random() * 30;
if (progress > 100) progress = 100;
progressBar.style.width = `${progress}%`;
progressText.textContent = `${Math.round(progress)}%`;
if (progress < 100) {
setTimeout(updateProgress, 200);
} else {
setTimeout(() => {
loading.style.display = 'none';
content.classList.add('show');
}, 500);
}
}
}
updateProgress();
});
3. スケルトンスクリーン型ローディング
See the Pen Untitled by ryoma (@hwjgdjpk-the-decoder) on CodePen.
HTML
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>スケルトンスクリーン型ローディング</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="loading" id="loading">
<div class="skeleton-container">
<div class="skeleton-header"></div>
<div class="skeleton-text"></div>
<div class="skeleton-text"></div>
<div class="skeleton-text short"></div>
</div>
</div>
<div class="content" id="content">
<h1>コンテンツが表示されました!</h1>
</div>
<script src="script.js"></script>
</body>
</html>
CSS
.loading {
padding: 20px;
}
.skeleton-container {
max-width: 600px;
margin: 0 auto;
}
.skeleton-header {
height: 40px;
background: linear-gradient(90deg, #f0f0f0 25%, #e0e0e0 50%, #f0f0f0 75%);
background-size: 200% 100%;
animation: loading 1.5s infinite;
border-radius: 4px;
margin-bottom: 20px;
}
.skeleton-text {
height: 20px;
background: linear-gradient(90deg, #f0f0f0 25%, #e0e0e0 50%, #f0f0f0 75%);
background-size: 200% 100%;
animation: loading 1.5s infinite;
border-radius: 4px;
margin-bottom: 10px;
}
.skeleton-text.short {
width: 70%;
}
@keyframes loading {
0% {
background-position: 200% 0;
}
100% {
background-position: -200% 0;
}
}
.content {
display: none;
}
.show {
display: block;
}
JavaScript
document.addEventListener('DOMContentLoaded', function() {
const loading = document.getElementById('loading');
const content = document.getElementById('content');
// ローディング画面を表示する関数
function showLoading() {
loading.style.display = 'flex';
content.classList.remove('show');
}
// ローディング画面を非表示にする関数
function hideLoading() {
loading.style.display = 'none';
content.classList.add('show');
}
// 3秒後にローディング画面を非表示にする
setTimeout(hideLoading, 3000);
});
さいごに
この記事ではJavaScriptでのローディングアニメーション実装について、基本から実践的な使用方法まで解説してきました。
主要なポイントは以下の通りです。
- 基本的なローディングアニメーションは、HTML、CSS、JavaScriptの連携で簡単に実装できる
- プログレスバーやカスタムアニメーションで、サイトの雰囲気に合わせたデザインが可能
- エラーハンドリングや互換性対応を忘れずに実装することが重要
簡単にサイトリッチに仕上げることができるので、ぜひ参考にしてみてください。
関連記事
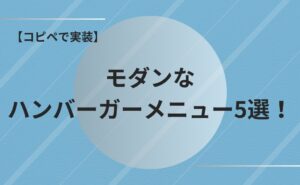
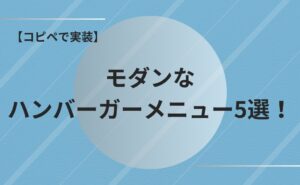
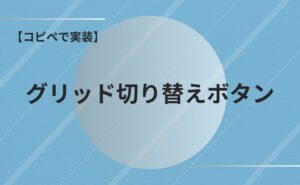
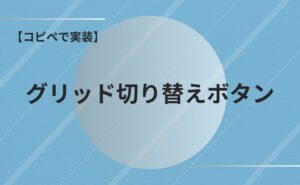